So, you’re diving into React JS, the JavaScript library that’s changing the game in front-end development. Whether you’re exploring React JS tutorials or brushing up on interview questions, understanding its component-based architecture and virtual DOM is key. W3Schools has useful documentation, and landing React JS jobs means showcasing your skills in projects. As a React JS developer, you’ll recognize the distinctive React JS logo everywhere. React JS vs React Native? That’s a conversation worth having.
Navigating the vast world of React JS can be overwhelming, but fear not! The React JS documentation, like a guiding light, provides clarity on architecture, animation, and API calls. Explore W3Schools for insightful tutorials, and consider alternatives for diverse solutions. Harness the power of JSX for dynamic UIs in single-page applications. Dive into React hooks and Native for seamless development. Education and treatment of children – handle your React components with care. State management, like a skilled conductor, orchestrates your app’s data symphony. Axios
Contents
Optimizing CSS for Dynamic and Reusable Styling in React
Normally big projects need it to be dynamic or have different reusable CSS classes that can be applied to multiple DOM elements, also there are some common CSS in two classes that can be separated as one common class and then apply multiple classes to each element where one is common CSS and one is element specific class. Here the need for multiple classes to a single element rises. Also, you can check about implementing material UI in React which is one of the demanding topics in React.
While working with HTML and CSS we encounter some situations where we need to apply more than one class to an element, i.e., applying one class for media queries and one class for color, size, and position to a div tag. Moreover, you can hire React developers from BOSC Tech Labs to help you apply these things and build complex React-based applications efficiently and correctly.
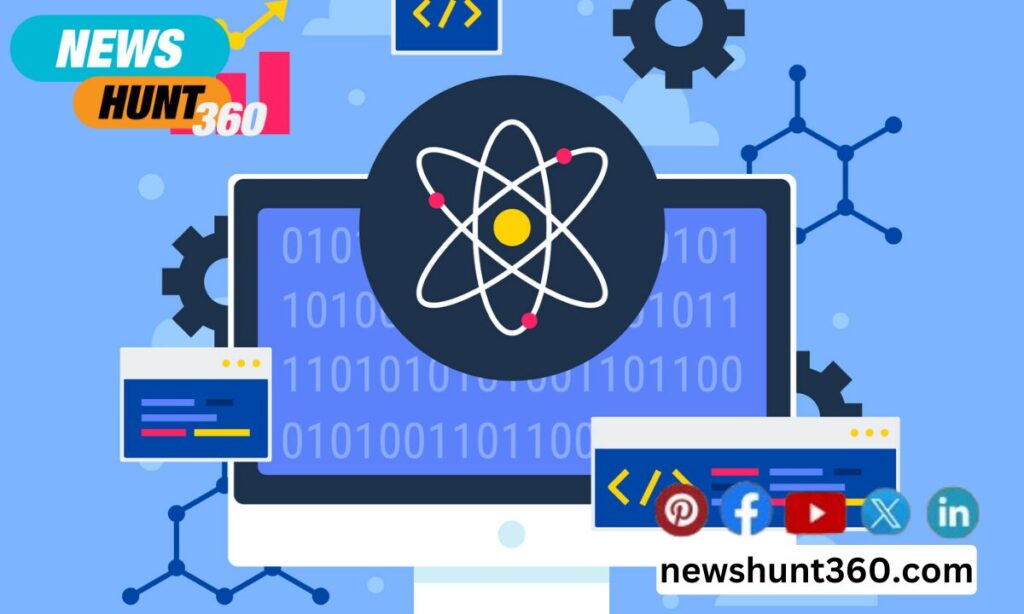
Mastering React JS: Guide to Declarative Programming and Advanced Techniques
React JS, a powerful web development framework, empowers developers with declarative programming. Dive into React JS basics with a comprehensive book PDF. Explore features like React Router, Flux architecture, and Redux. Enhance user experience with Bootstrap components, including buttons with onclick events. Elevate your skills, as Professional Development Schools embrace transformative technologies.
Are you ready to elevate your coding skills? Join a React JS Bootcamp and dive into React JS components, server-side rendering (SSR), and webpack. Brush up on basic interview questions and grab a React JS cheat sheet. Earn a React JS certification and ace coding tests with answers. Explore the power of immutable data and master Babel. Credential evaluation made easy!
For the above situation, we just write both the classes space separated to HTML element:
@media (max-width: 767px) { .hidden-mobile { display: none; } } .theme-format { color: ‘blue’; font-size: 16px; } <div class=”hidden-mobile theme-format”>SOME WEB CONTENT</div> |
Now, as we already know the React application has to have a Class component and a Functional component. So the keyword Class is already there for a class component in React, due to this we need to use className to tell React that the className is different from the class and className needs to be rendered inside an HTML tag as a CSS class.
Now to apply multiple classes we can just use space similar to HTML, i.e.,
function App() { return <div className=”class1 class2″>Normal Multiple Classes</div> } export default App |
Ways to Apply Multiple Classes to React DOM Element
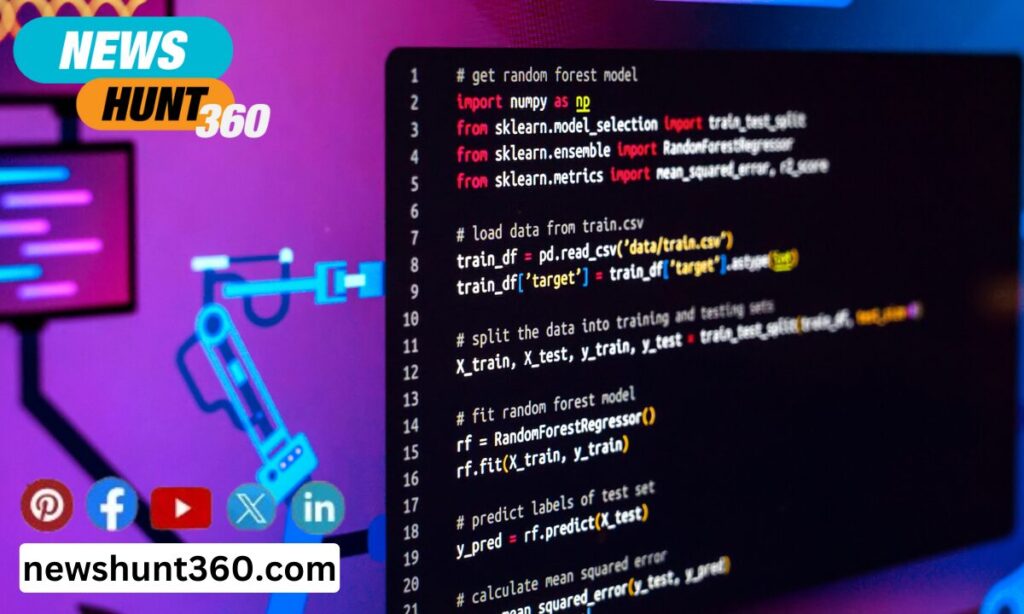
Static Classes:
As we have already seen above that is what static classes look like, there are a few more ways to apply static classes:
Static class with variable and then concatenation:
const varClass = “class1” <div className={varClass + ” ” + “class2”}>Normal Multiple Classes</div> // Or <div className={“class2 “.concat(varClass)}>Normal Multiple Classes</div> |
Similarly, we can apply classes combined as an array and then join it with space:
const arrClass = [“class1”, “class2”, “class3”] <div className={arrClass.join(“ “)}>Multiple Classes in array</div> |
Dynamic Classes:
The above classes are directly or indirectly static, but there are a few ways to add these classes dynamically. It can be with some condition from the parent component or even from the response from API.
Conditional classes are a widely used method as they give applications a different level of dynamicity from a front-end UI/UX perspective.
const [isWarning] = useState(true) return ( <> <div className={`box ${isWarning === true ? “warning” : “success”}`}> This is a message </div> </> ) |
Here the CSS can be:
.warning { color: ‘red’, } .success { color: ‘green’, } |
Classes as props from parent component:
const Child = props => { return <div className={`${props.status}`}>This is an error message</div> } function App() { return <Child statusClass=”error” /> } export default App |
Here the Child will get an error as a class given to that div element.
Using ClassNames Library:
All the methods above directly or indirectly force us to use space-separated classes, but the classNames library of React offers comma comma-separated class applying method.
Firstly install the library to your project by npm or yarn:
npm install classnames //Or yarn add classnames |
Now we can use it with all the above Static and dynamic methods but here the comma separation is needed instead of the space separation we used in the above examples i.e.,
import classNames from “classnames” import { useState } from “react” function App() { const [isWarning] = useState(true) return ( <div className={classNames(“box”, { warning: isWarning })}> This is a warning message </div> ) } export default App |
Here we applied two classes comma-separated, where one is Static, and the other one is conditional on one variable.
Conclusion
The examples above show that similar to normal HTML space separated by multiple classes, we can apply multiple classes to React DOM elements the same way with some additional possibilities like classes in array, variable, or even from another component’s props. There is one library that allows adding classes, just separating it with a comma as well.
In all the above ways there are endless possibilities of giving multiple classes statically or dynamically according to need and solving the needs of big projects.